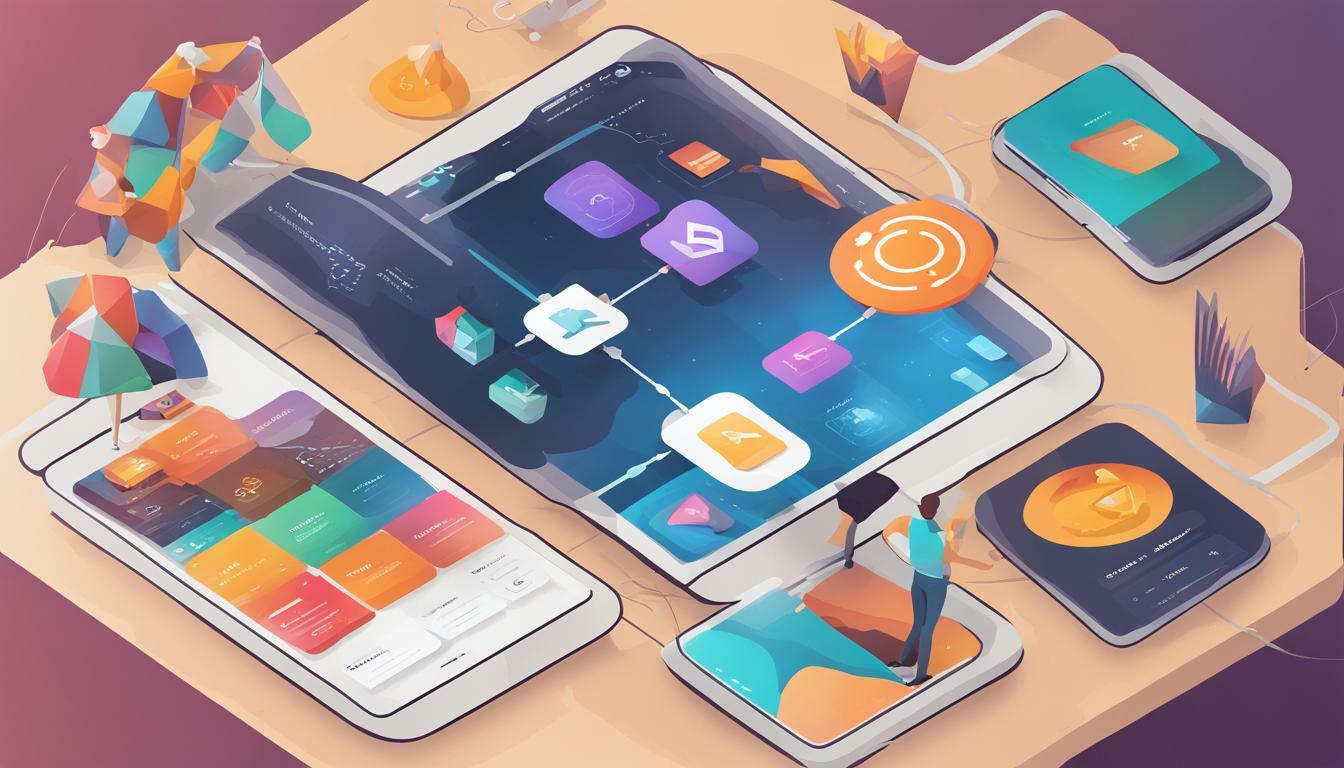
Welcome to our guide on Dart to Flutter mastery for seamless app development. As a mobile app developer, you understand the importance of creating high-quality, efficient, and user-friendly apps. That’s where mastering Dart programming language and Flutter framework come in. In this guide, we will highlight the key practices for becoming proficient in Dart to Flutter for seamless app development. You will learn the fundamentals of Dart programming language and the core concepts of the Flutter framework. We will explore the best practices for responsive UI design, efficient state management, performance optimization, and error handling. Additionally, we will dive into advanced techniques and features available in Flutter for app development. Lastly, we will provide insights into testing, debugging, and optimizing performance in Dart and Flutter. By the end of this guide, you will be equipped with the knowledge and skills to create top-notch mobile apps. Let’s get started with our Dart to Flutter Mastery: Key Practices for Seamless App Development guide!
Understanding Dart Language Fundamentals
Before diving into Flutter development, it’s important to have a solid understanding of the Dart programming language. Dart is a class-based, object-oriented language that was specifically designed for building web and mobile applications.
The syntax of Dart is similar to that of Java or C++, but with many modern features that make it easier to use. Dart has a dynamic type system, meaning that variable types are determined at runtime. It also has strong typing, where the type of a variable is checked at compile time to ensure that it is compatible with the operation being performed.
One of the key features of Dart is its support for asynchronous programming, which enables you to write code that can process multiple tasks at the same time. This is achieved using async/await keywords, which allow you to define tasks that can be executed concurrently.
The basic building blocks of any programming language are data types, variables, and functions. Dart provides a rich set of data types, including numbers, strings, booleans, lists, and maps. Variables in Dart can be declared using the var keyword or explicitly typed with the type annotation. Functions in Dart can be defined using the => operator or using the traditional method syntax with braces and a return statement.
Control Flow in Dart
Control flow structures are used to specify the order in which statements are executed in a program. Dart provides a range of control flow structures, including if-else statements, loops, and switches.
If-else statements allow you to execute different code blocks depending on whether a certain condition is true or false. Loops enable you to execute a block of code repeatedly, while switches allow you to match a value against a range of cases and execute the corresponding block of code.
When writing Dart code, it’s important to follow best practices to ensure your code is efficient, maintainable, and readable. Some tips for effective Dart programming include using descriptive variable and function names, avoiding excessive nesting of control flow statements, and using comments to document your code.
Exploring Flutter Framework Basics
The Flutter framework is a powerful tool for building beautiful, high-performance apps for mobile, web, and desktop. With Flutter, developers can use a single codebase to create apps for both Android and iOS, saving time and resources while ensuring consistency across platforms.
At the heart of the Flutter framework are widgets, which are building blocks for creating user interfaces. A widget can represent anything from a button or text field to a complex layout or animation. Flutter provides a wide range of pre-built widgets to choose from, as well as the ability to create custom widgets to fit specific needs.
Widgets and Layouts
Flutter widgets are designed to be highly customizable, with properties that can be adjusted to achieve the desired appearance and behavior. Many widgets are also designed to automatically adjust their size and layout based on the available space, making it easy to create responsive designs that look great on any screen size.
Layout is a critical aspect of app development, and Flutter provides a flexible system for organizing widgets on the screen. Developers can use pre-built layout widgets like Row and Column to arrange other widgets in a specific way, or create custom layouts using the Container widget.
Flutter also supports a number of other layout features, including alignment, padding, and the ability to layer widgets on top of each other using a Stack.
State Management
Another essential aspect of Flutter development is managing the state of the application. State refers to any data that can change over time and affect the user interface. For example, if an app displays a counter that increments every time a button is pressed, the current count is considered to be state.
Flutter provides several options for managing state, including StatefulWidget, which allows developers to create widgets that can change their appearance or behavior based on changes to their internal state. Flutter also provides a powerful mechanism called InheritedWidget, which allows widgets to access and share state data with their children widgets.
Best Practices for Project Structure
When starting a new project in Flutter, it’s important to establish a solid project structure to ensure that code is organized and easy to manage. Flutter provides some basic guidelines for organizing code into directories such as models, views, and controllers.
Developers can also take advantage of packages and plugins available through the Flutter community to add functionality to their apps more quickly and efficiently. It’s important to carefully evaluate any third-party packages before incorporating them into a project, however, to ensure they meet the project’s specific needs and are maintained by a reputable developer.
By understanding the basics of the Flutter framework and applying best practices for project organization, developers can create high-quality, efficient, and visually appealing apps for a variety of platforms.
Implementing App Development Best Practices
Implementing app development best practices is key to achieving mastery in Dart to Flutter app development. By incorporating these practices, developers can create efficient, high-quality, and user-friendly mobile applications. Here are some of the best practices that developers must consider:
Responsive UI Design
One of the most critical aspects of app development is creating a responsive UI design that adapts to different screen sizes and device orientations. Flutter makes this easier by providing built-in widgets that adjust to screen sizes and device orientations automatically. Developers can further optimize the UI by using layout widgets such as Column and Row, and by avoiding hard-coded values.
Efficient State Management
Effective state management is essential to developing scalable, maintainable, and performant Flutter apps. Flutter provides several tools for state management, including setState, InheritedWidget, and Provider. It’s important to choose the right state management approach based on the app’s complexity and requirements.
Code Reusability
Flutter is designed to promote code reuse, which reduces development time and effort while improving app quality. Developers can reuse code by creating custom widgets, separating UI from business logic, and using plugins and packages. Reusing code also makes it easier to maintain and update the app in the future.
Performance Optimization
Optimizing app performance is crucial for delivering a fast and smooth user experience. Developers can optimize performance by reducing app size, minimizing loading times, and optimizing UI rendering. Techniques such as lazy loading, code splitting, and caching can also help improve performance.
Error Handling
Error handling is an essential aspect of app development that ensures the app remains stable and functional. Flutter provides tools for handling errors and exceptions, such as try-catch blocks and onError callbacks. Developers must ensure the app is resilient to errors and crashes by testing and debugging thoroughly.
Advanced Techniques for Flutter App Development
Flutter is a powerful framework that offers a variety of advanced features and techniques for building high-quality mobile applications. Here are some of the top techniques that can take your Flutter app development to the next level:
1. Animations
Animating visual elements is an essential part of modern mobile app design. Flutter has a rich set of animation APIs that allow developers to create complex and beautiful animations with ease. The Animation class in Flutter provides a flexible and declarative way to define animations, and the AnimatedBuilder widget simplifies the process of integrating animations into your app.
2. Gesture Recognition
Flutter’s gesture recognition system enables developers to create apps that respond to user input in a natural and intuitive way. With GestureDetector, developers can detect a wide range of gestures, such as taps, swipes, and pinch-to-zoom. The DragTarget and DragSource widgets allow for advanced drag-and-drop interactions, while the LongPressDraggable widget adds a long-press gesture to the draggable functionality.
3. Networking
Mobile apps often need to communicate with remote servers to retrieve data or perform other tasks. Flutter provides a number of networking APIs that make it easy to send HTTP requests, handle responses, and work with RESTful APIs. The http package is a popular choice for making HTTP requests, while the Dio package offers additional features such as request cancellation and progress tracking.
4. Data Persistence
Flutter offers several options for storing data locally on the device. The shared_preferences package provides a simple way to store key-value pairs, while the sqflite package provides a more powerful SQL-based solution for local data storage. The Hive package offers an alternative to sqflite, providing a fast and easy-to-use NoSQL database for Flutter apps.
5. Integration with Native Device Features
Flutter apps can easily integrate with a wide variety of native device features, such as the camera, microphone, GPS, and more. The camera package provides a simple way to capture photos and videos, while the geolocator package offers a powerful API for working with location data. The flutter_blue package makes it easy to work with Bluetooth devices, and the package_info package provides information about the app itself, such as the version number and build number.
Incorporating these advanced techniques into your Flutter app development can help you create powerful and engaging mobile applications that stand out from the crowd. With a solid understanding of these features and techniques, you can take your app development skills to the next level and achieve Dart to Flutter Mastery!
Testing and Debugging in Dart and Flutter
As with any software development project, testing and debugging are essential parts of the mobile app development process. In Dart and Flutter, there are several tools and techniques that developers can use to ensure the quality and stability of their apps.
Testing Techniques in Dart and Flutter
Dart and Flutter offer several types of tests that developers can use to verify the functionality of their code:
- Unit tests: These tests check individual functions or classes in isolation to ensure they work as expected.
- Integration tests: These tests check the interaction between different parts of the app, such as user interface and data storage.
- Widget tests: These tests are used to verify the behavior of individual widgets in an app.
Developers can use built-in testing frameworks like flutter_test to create and run tests in Dart and Flutter projects. It’s essential to write effective tests that cover all possible cases and edge cases to ensure the stability of the app.
Debugging Tools and Techniques in Dart and Flutter
Debugging is the process of identifying and fixing issues in the code. Dart and Flutter provide several tools and techniques to help developers debug their code:
- Logging: Developers can use logging frameworks like dart:developer and flutter_logs to track the behavior of the app and identify issues.
- Debugging tools: Flutter provides built-in debugging tools like the Flutter DevTools and Flutter Inspector to help developers inspect and debug their apps.
- Hot reload: This feature in Flutter allows developers to quickly see the changes they make to the code without having to restart the app.
Developers can also use breakpoints and step-by-step debugging to identify and fix issues in the code. It’s essential to test the app thoroughly and fix any issues before releasing it to the users.
Optimizing Performance in Dart and Flutter
Optimizing performance is essential for creating high-quality mobile apps with Dart programming and Flutter development. By focusing on performance optimization strategies, developers can reduce app size and loading times, optimize UI rendering, and improve overall user experience.
Some of the key techniques for optimizing performance in Dart and Flutter include:
- Reducing app size through code optimization and minimizing resource usage
- Minimizing loading times by optimizing network requests and using caching
- Optimizing UI rendering through the use of Flutter widgets and efficient state management
- Optimizing database interactions and memory management
To identify and resolve performance bottlenecks in Flutter apps, developers can use performance profiling tools such as the Flutter Performance tab, which enables real-time tracking of app performance metrics. Additionally, developers can leverage best practices for performance optimization, such as reducing the number of unnecessary widgets and avoiding excessive widget rebuilds.
By implementing these performance optimization techniques and best practices in their Dart to Flutter projects, developers can create high-performing and efficient mobile apps that provide a seamless user experience.
Conclusion
Mastering Dart to Flutter is essential for seamless app development. By applying the key practices discussed in this article, developers can create high-quality, efficient, and user-friendly mobile applications that meet the needs of their users.
Remember to understand the fundamentals of the Dart language and explore the Flutter framework basics to create a solid foundation for your app development projects. Implement app development best practices and advanced techniques to take your Flutter apps to the next level.
Testing and debugging are critical components of the app development process, so take the time to learn effective testing techniques and use debugging tools to ensure the quality and stability of your Flutter apps. Finally, optimize performance by reducing app size, minimizing loading times, and optimizing UI rendering for a smooth user experience.
Start applying these key practices today and take your Dart to Flutter mastery to the next level. Happy app developing!